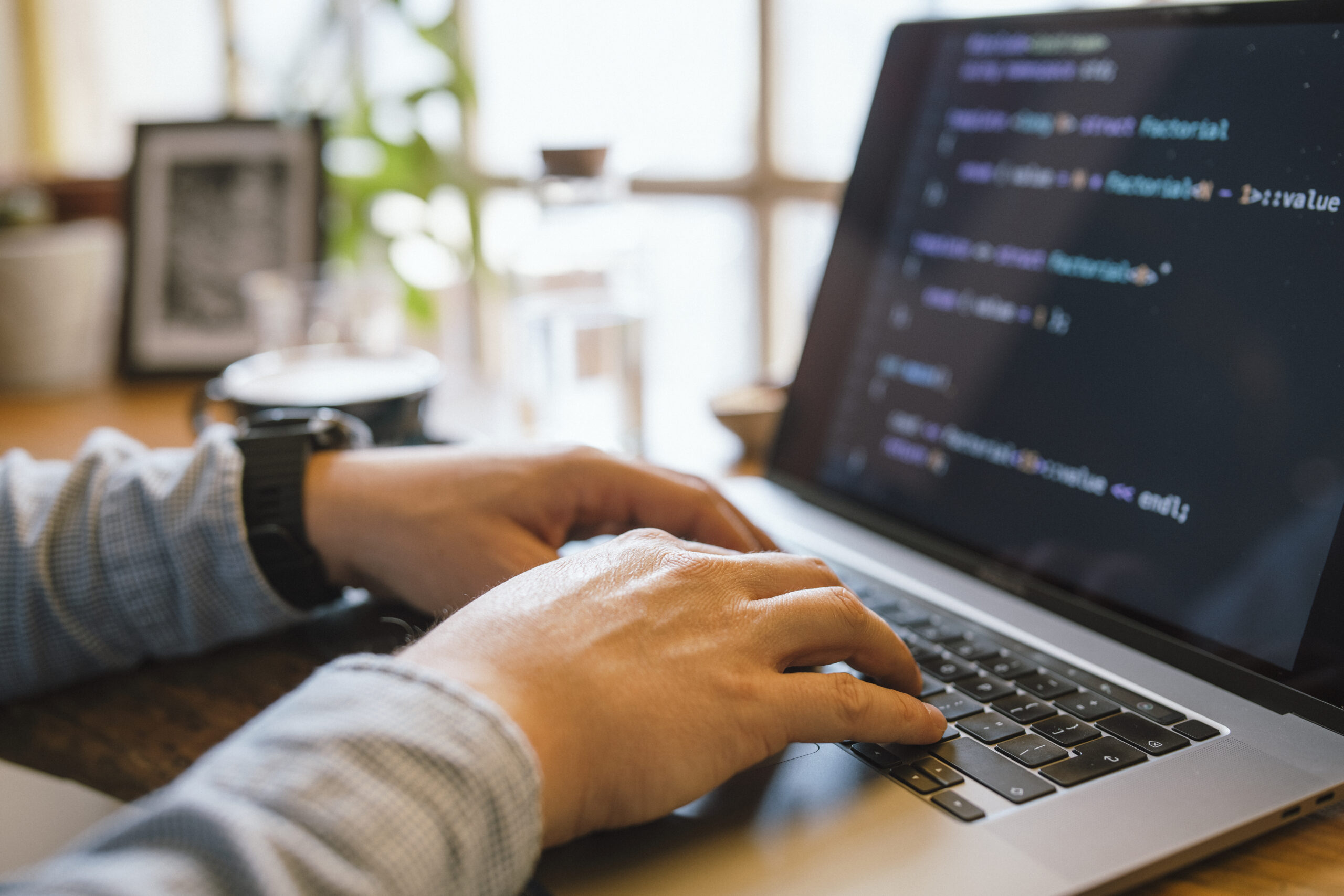
Debugging is Probably the most necessary — however usually neglected — techniques inside of a developer’s toolkit. It isn't just about fixing broken code; it’s about knowing how and why things go Incorrect, and Studying to Feel methodically to resolve difficulties proficiently. Whether you are a novice or perhaps a seasoned developer, sharpening your debugging expertise can preserve hrs of frustration and significantly boost your efficiency. Here i will discuss several methods that can help builders level up their debugging match by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of quickest ways builders can elevate their debugging skills is by mastering the tools they use everyday. When writing code is one Element of progress, being aware of the best way to interact with it successfully during execution is Similarly crucial. Modern enhancement environments appear equipped with impressive debugging abilities — but numerous builders only scratch the surface area of what these tools can perform.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources permit you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and perhaps modify code around the fly. When made use of correctly, they Enable you to notice specifically how your code behaves during execution, that's a must have for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclude developers. They let you inspect the DOM, observe community requests, view true-time performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can flip discouraging UI issues into manageable responsibilities.
For backend or program-level builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Regulate around jogging processes and memory administration. Understanding these tools could possibly have a steeper Understanding curve but pays off when debugging efficiency problems, memory leaks, or segmentation faults.
Past your IDE or debugger, come to be comfortable with version Regulate units like Git to understand code history, discover the exact instant bugs have been released, and isolate problematic improvements.
In the long run, mastering your tools implies likely further than default settings and shortcuts — it’s about developing an intimate familiarity with your enhancement setting to ensure that when difficulties crop up, you’re not shed at nighttime. The higher you already know your applications, the greater time you could expend resolving the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the situation
Among the most important — and infrequently disregarded — measures in successful debugging is reproducing the issue. Prior to leaping in to the code or creating guesses, builders have to have to make a steady atmosphere or scenario in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a sport of chance, normally leading to squandered time and fragile code adjustments.
Step one in reproducing a problem is accumulating as much context as possible. Inquire thoughts like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact disorders less than which the bug happens.
As you’ve collected enough facts, make an effort to recreate the problem in your neighborhood surroundings. This may suggest inputting a similar knowledge, simulating similar consumer interactions, or mimicking method states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the sting circumstances or state transitions concerned. These checks not only support expose the condition but additionally protect against regressions Later on.
From time to time, the issue could be natural environment-certain — it would materialize only on particular functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t only a phase — it’s a mentality. It demands persistence, observation, and a methodical method. But as soon as you can continuously recreate the bug, you might be currently halfway to fixing it. Which has a reproducible scenario, You should use your debugging equipment far more proficiently, exam opportunity fixes properly, and talk a lot more Obviously along with your group or consumers. It turns an abstract complaint into a concrete problem — and that’s exactly where developers thrive.
Study and Realize the Error Messages
Error messages are frequently the most useful clues a developer has when anything goes Completely wrong. Rather then looking at them as discouraging interruptions, builders really should study to deal with error messages as direct communications within the system. They usually tell you what exactly occurred, in which it happened, and sometimes even why it transpired — if you understand how to interpret them.
Start off by examining the concept diligently As well as in entire. Several developers, specially when beneath time force, look at the very first line and straight away start earning assumptions. But further while in the error stack or logs may possibly lie the correct root trigger. Don’t just duplicate and paste error messages into serps — read through and comprehend them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic error? Does it position to a specific file and line range? What module or purpose triggered it? These thoughts can tutorial your investigation and stage you towards the accountable code.
It’s also helpful to be aware of the terminology of the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java generally abide by predictable designs, and Studying to acknowledge these can significantly hasten your debugging process.
Some problems are imprecise or generic, As well as in These instances, it’s critical to look at the context by which the mistake happened. Check out related log entries, enter values, and up to date variations inside the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede more substantial difficulties and provide hints about probable bugs.
Eventually, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, aiding you pinpoint troubles a lot quicker, lessen debugging time, and turn into a additional efficient and confident developer.
Use Logging Wisely
Logging is Just about the most highly effective applications within a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood with no need to pause execution or stage with the code line by line.
A fantastic logging system starts off with figuring out what to log and at what stage. Widespread logging levels include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details in the course of advancement, Details for normal functions (like prosperous start off-ups), WARN for prospective problems that don’t break the applying, Mistake for genuine troubles, and FATAL in the event the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. Too much logging can obscure vital messages and decelerate your method. Focus on vital gatherings, state variations, enter/output values, and critical selection points in the code.
Format your log messages Evidently and regularly. Involve context, for example timestamps, ask for IDs, and function names, so it’s easier to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a properly-thought-out logging solution, you are able to decrease the time it takes to spot troubles, attain deeper visibility into your programs, and Increase the overall maintainability and trustworthiness of your code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a sort of investigation. To correctly determine and take care of bugs, developers should technique the procedure similar to a detective solving a mystery. This frame of mind can help break down sophisticated troubles into workable pieces and follow clues logically to uncover the root result in.
Commence by collecting evidence. Think about the symptoms of the trouble: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect as much related facts as you may devoid of leaping to conclusions. Use logs, test instances, and user reports to piece together a transparent photograph of what’s happening.
Next, form hypotheses. Ask yourself: What could be producing this actions? Have any improvements not long ago been manufactured into the codebase? Has this difficulty transpired just before below similar instances? The target is usually to slim down choices and identify potential culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge inside a managed natural environment. In case you suspect a particular function or part, isolate it and verify if The problem persists. Similar to a detective conducting interviews, question your code concerns Developers blog and Enable the outcome lead you nearer to the truth.
Fork out close focus to small facts. Bugs usually disguise while in the least predicted places—just like a missing semicolon, an off-by-just one error, or a race affliction. Be comprehensive and affected individual, resisting the urge to patch the issue devoid of totally being familiar with it. Short term fixes may perhaps conceal the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you experimented with and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for future concerns and assistance Other people recognize your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering concealed difficulties in complex techniques.
Produce Checks
Writing tests is one of the best solutions to improve your debugging capabilities and In general development efficiency. Tests not just support capture bugs early and also function a security Web that offers you confidence when creating adjustments to the codebase. A properly-examined software is simpler to debug as it lets you pinpoint particularly wherever and when a dilemma occurs.
Start with unit tests, which focus on individual capabilities or modules. These compact, isolated checks can immediately expose whether a specific piece of logic is Operating as expected. When a exam fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Device tests are especially useful for catching regression bugs—challenges that reappear immediately after Formerly becoming fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These assistance be sure that different parts of your software operate with each other effortlessly. They’re specially beneficial for catching bugs that occur in elaborate programs with a number of components or products and services interacting. If anything breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically about your code. To check a function thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This amount of understanding Obviously leads to higher code composition and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the exam fails regularly, you may focus on repairing the bug and enjoy your test go when The difficulty is settled. This tactic ensures that precisely the same bug doesn’t return Down the road.
In short, composing checks turns debugging from the irritating guessing match right into a structured and predictable process—serving to you capture more bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging a tough concern, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Option just after Alternative. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new standpoint.
If you're far too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this state, your brain gets to be less efficient at trouble-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–quarter-hour can refresh your aim. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially through more time debugging sessions. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping absent means that you can return with renewed Vitality and a clearer way of thinking. You could possibly all of a sudden detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
Should you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in speedier and more effective debugging Eventually.
In short, using breaks is not really a sign of weak point—it’s a sensible approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your progress. Debugging is usually a mental puzzle, and relaxation is part of fixing it.
Understand From Each individual Bug
Just about every bug you encounter is much more than just A brief setback—It is really an opportunity to expand being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something beneficial should you make the effort to reflect and evaluate what went Improper.
Start off by inquiring on your own a handful of key concerns once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device screening, code testimonials, or logging? The responses normally expose blind places as part of your workflow or knowledge and assist you Establish much better coding patterns transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and what you learned. Eventually, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with your friends could be Particularly powerful. Irrespective of whether it’s through a Slack concept, a short generate-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts staff efficiency and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your way of thinking from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who generate excellent code, but those who continually learn from their problems.
In the end, Every single bug you fix adds a completely new layer in your skill set. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, far more able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — although the payoff is large. It helps make you a far more economical, self-confident, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at what you do.