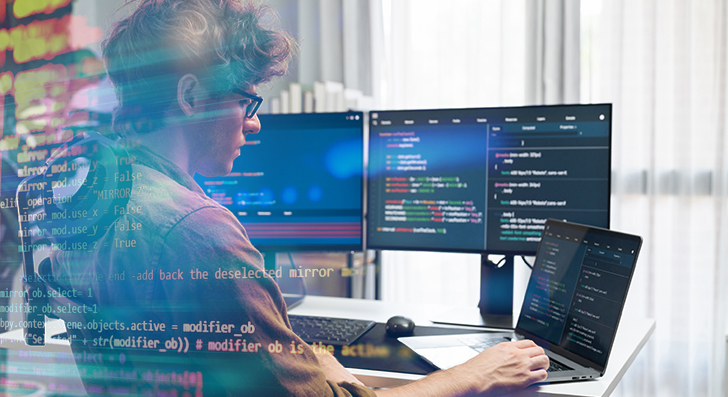
Scalability usually means your application can manage development—more people, a lot more information, and much more traffic—without the need of breaking. For a developer, creating with scalability in your mind will save time and tension afterwards. Right here’s a transparent and functional manual to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your program from the start. Several purposes fail every time they expand speedy since the first style can’t cope with the extra load. Being a developer, you might want to Feel early regarding how your method will behave under pressure.
Get started by developing your architecture being flexible. Keep away from monolithic codebases in which all the things is tightly related. Instead, use modular structure or microservices. These patterns split your application into smaller, independent components. Just about every module or service can scale on its own with no influencing the whole method.
Also, contemplate your databases from working day just one. Will it need to deal with 1,000,000 people or just a hundred? Choose the proper kind—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different vital point is in order to avoid hardcoding assumptions. Don’t produce code that only is effective less than current conditions. Consider what would take place Should your consumer base doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or event-pushed units. These assistance your app handle more requests without getting overloaded.
When you Establish with scalability in your mind, you are not just getting ready for success—you're lowering potential head aches. A effectively-planned method is easier to take care of, adapt, and improve. It’s superior to get ready early than to rebuild afterwards.
Use the appropriate Database
Choosing the right databases can be a crucial part of setting up scalable apps. Not all databases are created precisely the same, and using the Incorrect you can sluggish you down or perhaps cause failures as your application grows.
Start off by knowing your information. Is it highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are generally powerful with interactions, transactions, and consistency. They also aid scaling approaches like go through replicas, indexing, and partitioning to handle more website traffic and info.
In the event your knowledge is more versatile—like person activity logs, product or service catalogs, or documents—take into account a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured data and may scale horizontally much more quickly.
Also, think about your examine and write designs. Are you presently carrying out numerous reads with fewer writes? Use caching and read replicas. Do you think you're handling a weighty generate load? Consider databases that could tackle higher compose throughput, or maybe event-primarily based knowledge storage devices like Apache Kafka (for short-term knowledge streams).
It’s also clever to think ahead. You may not want State-of-the-art scaling options now, but choosing a database that supports them indicates you received’t have to have to modify afterwards.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And generally observe database efficiency while you expand.
In a nutshell, the best database is dependent upon your app’s construction, speed wants, And the way you be expecting it to improve. Acquire time to select correctly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, every small hold off provides up. Inadequately composed code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish successful logic from the start.
Begin by composing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all pointless. Don’t pick the most advanced Remedy if a simple a person performs. Keep your functions shorter, centered, and easy to check. Use profiling equipment to find bottlenecks—sites the place your code will take too very long to run or utilizes too much memory.
Following, take a look at your databases queries. These frequently gradual items down more than the code by itself. Make certain Just about every query only asks for the information you really need. Keep away from SELECT *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And steer clear of undertaking a lot of joins, Specifically throughout large tables.
For those who recognize a similar information staying requested time and again, use caching. Store the final results temporarily working with tools like Redis or Memcached and that means you don’t really have to repeat high-priced functions.
Also, batch your databases operations once you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application extra efficient.
Remember to examination with massive datasets. Code and queries that function fantastic with one hundred documents could possibly crash when they have to deal with 1 million.
In a nutshell, scalable applications are rapidly applications. Keep the code limited, your queries lean, and use caching when needed. These techniques enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of more customers and much more site visitors. If almost everything goes by way of a person server, it will rapidly become a bottleneck. That’s exactly where load balancing and caching come in. These two applications help keep the application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic throughout several servers. In lieu of just one server executing every one of the operate, the load balancer routes consumers to distinct servers depending on availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Other people. Tools like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it might be reused speedily. When customers ask for the exact same details again—like an item website page or perhaps a profile—you don’t have to fetch it from the databases each and every time. You can provide it in the cache.
There's two frequent types of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Shopper-side caching (like browser caching or CDN caching) suppliers static information near the consumer.
Caching cuts down database load, increases speed, and would make your app additional effective.
Use caching for things which don’t alter generally. And usually make sure your cache is up to date when details does modify.
To put it briefly, load balancing and caching are easy but highly effective resources. Jointly, they assist your app take care of more consumers, keep fast, and Recuperate from challenges. If you intend to improve, you'll need equally.
Use Cloud and Container Applications
To construct scalable apps, you would like tools that allow your application improve easily. That’s exactly where cloud platforms and containers can be found in. They offer you adaptability, reduce set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services more info as you would like them. You don’t have to purchase hardware or guess potential ability. When website traffic boosts, you could increase more assets with just a couple clicks or routinely working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also offer you expert services like managed databases, storage, load balancing, and protection equipment. It is possible to target constructing your app as an alternative to controlling infrastructure.
Containers are One more essential Software. A container offers your application and almost everything it should run—code, libraries, settings—into a person device. This can make it effortless to move your app concerning environments, from the laptop computer towards the cloud, with out surprises. Docker is the preferred Resource for this.
When your application employs several containers, tools like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If one section of the app crashes, it restarts it quickly.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale parts independently, that is perfect for functionality and reliability.
Briefly, making use of cloud and container applications signifies you are able to scale speedy, deploy simply, and Recuperate immediately when troubles occur. If you'd like your application to develop without the need of limitations, get started applying these resources early. They help save time, decrease possibility, and assist you to keep centered on developing, not repairing.
Monitor Almost everything
If you don’t keep an eye on your software, you won’t know when items go Erroneous. Checking aids the thing is how your application is performing, place challenges early, and make much better choices as your application grows. It’s a critical part of creating scalable devices.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this information.
Don’t just check your servers—keep an eye on your application too. Keep an eye on how long it will take for consumers to load webpages, how often problems transpire, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening inside your code.
Set up alerts for important problems. For instance, In case your response time goes above a Restrict or simply a company goes down, you need to get notified instantly. This assists you repair issues fast, often right before buyers even detect.
Checking is additionally helpful when you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you could roll it back again just before it causes serious hurt.
As your app grows, traffic and data maximize. With no monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper applications in position, you stay on top of things.
In brief, checking will help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t just for significant organizations. Even small apps have to have a powerful Basis. By creating diligently, optimizing properly, and utilizing the right equipment, you can Create applications that expand effortlessly with out breaking stressed. Start tiny, Assume large, and Create good.